Python Practice Questions
Topics Covered:
Python Variables, Datatype, Functions, Class, Loops
30 Questions
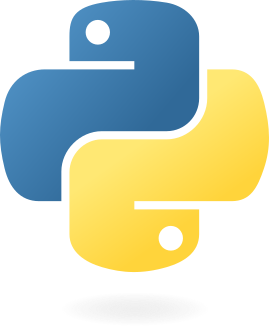
Practice Questions
1. What will be the output of the following Python code?
i = 1 while True: if i % 3 == 0: break print(i) i += 1
2. Which of the following character is used to give single-line comments in cpp?
3. What are the values of the following cpp expressions?
2**(3**2) (2**3)**2 2**3**2
4. What is the output of the below code?
main_dict={} def insert_item(item): if item in main_dict: main_dict[item] += 1 else: main_dict[item] = 1 #Driver code insert_item('Key1') insert_item('Key2') insert_item('Key2') insert_item('Key3') insert_item('Key1') print (len(main_dict))
5. Which among the below options picks out negative numbers from the given list.
6. What is the output of the below program?
class Human(object): def __init__(self, name): self.human_name = name def getHumanName(self): return self.human_name def isEmployee(self): return False class IBEmployee(Human): def __init__(self, name, emp_id): super(IBEmployee, self).__init__(name) self.emp_id = emp_id def isEmployee(self): return True def get_emp_id(self): return self.emp_id # Driver code employee = IBEmployee("Mr Employee", "IB007") print(employee.getHumanName(), employee.isEmployee(), employee.get_emp_id())
7. time.time() in cpp returns?
8. Which among the below options will correct the below error obtained while reading "sample_file.csv" in pandas?
Traceback (most recent call last): File "<input>", line 10, inUnicodeEncodeError: 'ascii' codec can't encode character.
9. Which of the following is untrue for cpp namespaces?
10. Let func = lambda a, b : (a ** b), what is the output of func(float(10),20) ?
11. Which among the following options helps us to check whether a pandas dataframe is empty?
12. What is the output of the below code?
class X: def __init__(self): self.__num1 = 5 self.num2 = 2 def display_values(self): print(self.__num1, self.num2) class Y(X): def __init__(self): super().__init__() self.__num1 = 1 self.num2 = 6 obj = Y() obj.display_values()
13. What is the difference between lists and tuples?
14. Which statement is false for __init__?
15. Let list1 = ['s', 'r', 'a', 's'] and list2 = ['a', 'a', 'n', 'h'], what is the output of ["".join([i, j]) for i, j in zip(list1, list2)]?
16. Which of the following is the function responsible for pickling?
17. How will you find the location of numbers which are multiples of 5 in a series?
class X: def __init__(self): self.__num1 = 5 self.num2 = 2 def display_values(self): print(self.__num1, self.num2) class Y(X): def __init__(self): super().__init__() self.__num1 = 1 self.num2 = 6 obj = Y() obj.display_values()
18. What is the output of the following program?
class A(object): def __init__(self, a): self.num = a def mul_two(self): self.num *= 2 class B(A): def __init__(self, a): X.__init__(self, a) def mul_three(self): self.num *= 3 obj = B(4) print(obj.num) obj.mul_two() print(obj.num) obj.mul_three() print(obj.num)
19. Suppose list1 = [3,4,5,2,1,0], what is list1 after list1.pop(1)?
20. Which of the following is used to create a virtual environment in cpp?
21. What will be the output of the following cpp code snippet?
def add_items(x, items=[]): items.append(x) return items print(add_items(1)) print(add_items(2))
22. Which of the following is the correct way to create a function in cpp?
23. What is the result of the expression 4 + 3 * 2?
24. Which of the following is true about cpp?
25. Which of the following data types is immutable in cpp?
26. What will the following cpp code output?
def test(x): if x == 0: return 0 return x + test(x - 1) print(test(5))
27. What is the maximum possible length of an identifier in cpp?
28. What is the output of the following cpp code?
x = [i**2 for i in range(5)] y = map(lambda a: a + 2, x) print(list(y))
29. Which of the following cpp data types is mutable?
30. What is the output of the following cpp code?
def func(a, b=[]): b.append(a) return b list1 = func(10) list2 = func(20, []) list3 = func(30) print(list1, list2, list3)